Java Variables
Table of Contents
1. What is Variables?
Object stores its state in fields.
int speed = 0; int gear = 1;
2. Different Kinds of Variables in Java
The Java programming language defines the following kinds of variables:
-
Instance Variables (Non-Static Fields)
Objects store their individual states in "non-static fields", that is, fields declared without the static keyword.
Non-static fields are also known as instance variables because their values are unique to each instance of a class (to each object, in other words); the currentSpeed of one bicycle is independent from the currentSpeed of another.
-
Class Variables (Static Fields)
A class variable is any field declared with the static modifier; this tells the compiler that there is exactly one copy of this variable in existence, regardless of how many times the class has been instantiated.
A field defining the number of gears for a particular kind of bicycle could be marked as static since conceptually the same number of gears will apply to all instances.
The code static int numGears = 6; would create such a static field. Additionally, the keyword final could be added to indicate that the number of gears will never change.
-
Local Variables
Similar to how an object stores its state in fields, a method will often store its temporary state in local variables.
The syntax for declaring a local variable is similar to declaring a field (for example, int count = 0;). There is no special keyword designating a variable as local; that determination comes entirely from the location in which the variable is declared — which is between the opening and closing braces of a method.
As such, local variables are only visible to the methods in which they are declared; they are not accessible from the rest of the class.
-
Parameters
The main method is public static void main(String[] args). Here, the args variable is the parameter to this method. The important thing to remember is that parameters are always classified as "variables" not "fields".
Parameters are similar to local variable, Parameters are only visible to the methods in which they are passed; they are not accessible from the rest of the class.
3. Java Variable Naming Conventions
The rules and conventions for naming your variables can be summarized as follows:
-
Variable names are case-sensitive. A variable's name can be any legal identifier — an unlimited-length sequence of letters, digits, dollar signs "$", or underscore characters "_", beginning with a letter, the dollar sign "$", or the underscore character "_".
-
The convention, however, is to always begin your variable names with a letter, not "$" or "_". Additionally, the dollar sign character, by convention, is never used at all. A similar convention exists for the underscore character; while it's technically legal to begin your variable's name with "_", this practice is discouraged. White space is not permitted.
-
Subsequent characters may be letters, digits, dollar signs, or underscore characters.
-
If the name you choose consists of only one word, spell that word in all lowercase letters. If it consists of more than one word, capitalize the first letter of each subsequent word. The names gearRatio and currentGear are prime examples of this convention.
-
If your variable stores a constant value, such as static final int NUM_GEARS = 6, the convention changes slightly, capitalizing every letter and separating subsequent words with the underscore character. By convention, the underscore character is never used elsewhere.
4. Primitive Data Types
A primitive type is predefined by the language and is named by a reserved keyword. Primitive values do not share state with other primitive values. The eight primitive data types supported by the Java programming language are:
-
byte
The byte data type is an 8-bit signed two's complement integer.
It has a minimum value of -128 and a maximum value of 127 (inclusive).
Default Value - 0
Example : byte a = 100;
-
short
The short data type is a 16-bit signed two's complement integer.
It has a minimum value of -32,768 and a maximum value of 32,767 (inclusive).
Default Value - 0
Example : short s = 10000;
-
int
By default, the int data type is a 32-bit signed two's complement integer.
It has a minimum value of -231 and a maximum value of 231-1.
Default Value - 0
Example : int a = 100000;
-
long
The long data type is a 64-bit two's complement integer.
The signed long has a minimum value of -263 and a maximum value of 263-1.
Default Value – 0L
Example : long a = 100000L;
-
float
The float data type is a single-precision 32-bit IEEE 754 floating point.
The minimum/maximum value of float is not the same as that of the int data type (despite both being made of 32-bits). The full range of float values is beyond the scope of this tutorial. For now, the only thing you need to know is that you’ll use float (and double – see below) for saving decimal values.
Default Value – 0.0f
Example : float f1 = 234.5f;
-
double
The double data type is a double-precision 64-bit IEEE 754 floating point.
As with float, discussing the minimum/maximum value of double data type is beyond the scope of this article. What you should know is that double is a much more precise type than float. For all practical purposes, it is recommended that you use double instead of float for storing decimal values.
Default Value – 0.0d
Example : double d1 = 123.4;
-
boolean
The boolean data type has only two possible values: true and false.
Use this data type for simple flags that track true/false conditions.
This data type represents one bit of information, but its "size" isn't something that's precisely defined.
Default Value - false
Example : boolean one = true;
-
char
The char data type is a single 16-bit Unicode character.
It has a minimum value of '\u0000' (or 0) and a maximum value of '\uffff' (or 65,535 inclusive).
Default Value - '\u0000'
Example : char letterA ='A';
In addition to the eight primitive data types listed above, the Java programming language also provides special support for character strings via the java.lang.String class. Enclosing your character string within double quotes will automatically create a new String object; for example, String s = "this is a string";. String objects are immutable, which means that once created, their values cannot be changed. The String class is not technically a primitive data type, but considering the special support given to it by the language, you'll probably tend to think of it as such.
Local variables are slightly different; the compiler never assigns a default value to an uninitialized local variable. If you cannot initialize your local variable where it is declared, make sure to assign it a value before you attempt to use it. Accessing an uninitialized local variable will result in a compile-time error.
5. Arrays
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created.
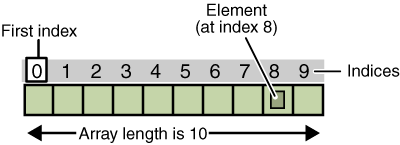
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the preceding illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8.
Declaring a Variable to Refer to an Array
// declares an array of integers int[] anArray;
Like declarations for variables of other types, an array declaration has two components: the array's type and the array's name.
-
An array's type is written as type[], where type is the data type of the contained elements; the brackets are special symbols indicating that this variable holds an array. The size of the array is not part of its type (which is why the brackets are empty).
-
An array's name can be anything you want, provided that it follows the rules and conventions as previously discussed in the naming section. As with variables of other types.
The declaration does not actually create an array; it simply tells the compiler that this variable will hold an array of the specified type.
Similarly, you can declare arrays of other types (byte, char, long etc)
You can also place the brackets after the array's name:
// this form is discouraged float anArrayOfFloats[];
However, convention discourages this form; the brackets identify the array type and should appear with the type designation.
Creating an Array
One way to create an array is with the new operator.
// create an array of integers anArray = new int[10];
Alternatively, you can use the shortcut syntax to create and initialize an array:
int[] anArray = { 100, 200, 300, 400, 500, 600, 700, 800, 900, 1000 };
Here the length of the array is determined by the number of values provided between braces and separated by commas.
Initializing an Array
The next few lines assign values to each element of the array:
anArray[0] = 100; // initialize first element anArray[1] = 200; // initialize second element anArray[2] = 300; // and so forth
Accessing an Array
Each array element is accessed by its numerical index:
System.out.println("Element 1 at index 0: " + anArray[0]); System.out.println("Element 2 at index 1: " + anArray[1]); System.out.println("Element 3 at index 2: " + anArray[2]);
Example for an Array
/** * A class used to demonstrate usage of Array. * * @author Vasanth * */ public class ArrayDemo { /** * Main. * * @param args */ public static void main(String[] args) { // declares an array of integers int[] anArray; // allocates memory for 10 integers anArray = new int[10]; // initialize first element anArray[0] = 100; // initialize second element anArray[1] = 200; // and so forth anArray[2] = 300; anArray[3] = 400; anArray[4] = 500; anArray[5] = 600; anArray[6] = 700; anArray[7] = 800; anArray[8] = 900; anArray[9] = 1000; System.out.println("Element at index 0: " + anArray[0]); System.out.println("Element at index 1: " + anArray[1]); System.out.println("Element at index 2: " + anArray[2]); System.out.println("Element at index 3: " + anArray[3]); System.out.println("Element at index 4: " + anArray[4]); System.out.println("Element at index 5: " + anArray[5]); System.out.println("Element at index 6: " + anArray[6]); System.out.println("Element at index 7: " + anArray[7]); System.out.println("Element at index 8: " + anArray[8]); System.out.println("Element at index 9: " + anArray[9]); } }
Output for the program is
Element at index 0: 100 Element at index 1: 200 Element at index 2: 300 Element at index 3: 400 Element at index 4: 500 Element at index 5: 600 Element at index 6: 700 Element at index 7: 800 Element at index 8: 900 Element at index 9: 1000
Multi-Dimensional Array
The arrays you have been using so far have only held one column of data. But you can set up an array to hold more than one column. These are called multi-dimensional arrays.
As an example, think of a spreadsheet with rows and columns. If you have 3 rows and 3 columns then your spreadsheet can hold 9 numbers. It might look like this:
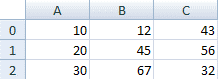
Example for an Multi-Dimensional Array.
/** * A class used to demonstrate usage of Multi-Dimensional Array. * * @author Vasanth * */ public class MultiDimensionalArrayDemo { /** * Main. * * @param args */ public static void main(String[] args) { // declares an multi-dimensional array of integers int[][] anMultiArray; // create a multi-dimensional array of 3*3 anMultiArray = new int[3][3]; // initialize multi-dimensional array // initialize first row. anMultiArray[0][0] = 10; anMultiArray[0][1] = 12; anMultiArray[0][2] = 43; // initialize second row. anMultiArray[1][0] = 20; anMultiArray[1][1] = 45; anMultiArray[1][2] = 56; // initialize third row. anMultiArray[2][0] = 30; anMultiArray[2][1] = 67; anMultiArray[2][2] = 32; // Accessing multi-dimensional array. System.out.println("Element at row 0 col 0: " + anMultiArray[0][0]); System.out.println("Element at row 0 col 1: " + anMultiArray[0][1]); System.out.println("Element at row 0 col 2: " + anMultiArray[0][2]); System.out.println("Element at row 1 col 0: " + anMultiArray[1][0]); System.out.println("Element at row 1 col 1: " + anMultiArray[1][1]); System.out.println("Element at row 1 col 2: " + anMultiArray[1][2]); System.out.println("Element at row 2 col 0: " + anMultiArray[2][0]); System.out.println("Element at row 2 col 1: " + anMultiArray[2][1]); System.out.println("Element at row 2 col 2: " + anMultiArray[2][2]); } }
Output for the program is
Element at row 0 col 0: 10 Element at row 0 col 1: 12 Element at row 0 col 2: 43 Element at row 1 col 0: 20 Element at row 1 col 1: 45 Element at row 1 col 2: 56 Element at row 2 col 0: 30 Element at row 2 col 1: 67 Element at row 2 col 2: 32
In the Java programming language, a multidimensional array is an array whose components are themselves arrays. This is unlike arrays in C or Fortran. A consequence of this is that the rows are allowed to vary in length, as shown in the following MultiDimArrayDemo program:
public class MultiDimArrayDemo { public static void main(String[] args) { String[][] names = { { "Mr. ", "Mrs. ", "Ms. " }, { "Smith", "Jones" } }; // Mr. Smith System.out.println(names[0][0] + names[1][0]); // Ms. Jones System.out.println(names[0][2] + names[1][1]); } }
Output for the program is
Mr. Smith Ms. Jones
No comments:
Post a Comment